Note
Go to the end to download the full example code
Cube & Slider Control#
This example shows how to use the UI API. We will demonstrate how to create a cube and control with sliders.
First, some imports.
import numpy as np
import fury
First we need to fetch some icons that are included in FURY.
fury.data.fetch_viz_icons()
Data size is approximately 12KB
Dataset is already in place. If you want to fetch it again please first remove the folder /Users/skoudoro/.fury/icons
({'icomoon.tar.gz': ('https://digital.lib.washington.edu/researchworks/bitstream/handle/1773/38478/icomoon.tar.gz', 'BC1FEEA6F58BA3601D6A0B029EB8DFC5F352E21F2A16BA41099A96AA3F5A4735')}, '/Users/skoudoro/.fury/icons')
Cube and sliders#
Add a cube to the scene .
Now we’ll add five sliders: 1 circular and 4 linear sliders. By default the alignments are ‘bottom’ for horizontal and ‘top’ for vertical.
ring_slider = fury.ui.RingSlider2D(
center=(630, 400), initial_value=0, text_template="{angle:5.1f}°"
)
hor_line_slider_text_top = fury.ui.LineSlider2D(
center=(400, 230),
initial_value=0,
orientation="horizontal",
min_value=-10,
max_value=10,
text_alignment="top",
)
hor_line_slider_text_bottom = fury.ui.LineSlider2D(
center=(400, 200),
initial_value=0,
orientation="horizontal",
min_value=-10,
max_value=10,
text_alignment="bottom",
)
ver_line_slider_text_left = fury.ui.LineSlider2D(
center=(100, 400),
initial_value=0,
orientation="vertical",
min_value=-10,
max_value=10,
text_alignment="left",
)
ver_line_slider_text_right = fury.ui.LineSlider2D(
center=(150, 400),
initial_value=0,
orientation="vertical",
min_value=-10,
max_value=10,
text_alignment="right",
)
We can use a callback to rotate the cube with the ring slider.
def rotate_cube(slider):
angle = slider.value
previous_angle = slider.previous_value
rotation_angle = angle - previous_angle
cube.RotateX(rotation_angle)
ring_slider.on_change = rotate_cube
Similarly, we can translate the cube with the line slider.
def translate_cube_ver(slider):
value = slider.value
cube.SetPosition(0, value, 0)
def translate_cube_hor(slider):
value = slider.value
cube.SetPosition(value, 0, 0)
hor_line_slider_text_top.on_change = translate_cube_hor
hor_line_slider_text_bottom.on_change = translate_cube_hor
ver_line_slider_text_left.on_change = translate_cube_ver
ver_line_slider_text_right.on_change = translate_cube_ver
Show Manager#
Now that all the elements have been initialised, we add them to the show manager.
current_size = (800, 800)
show_manager = fury.window.ShowManager(size=current_size, title="FURY Cube Example")
show_manager.scene.add(cube)
show_manager.scene.add(ring_slider)
show_manager.scene.add(hor_line_slider_text_top)
show_manager.scene.add(hor_line_slider_text_bottom)
show_manager.scene.add(ver_line_slider_text_left)
show_manager.scene.add(ver_line_slider_text_right)
Visibility by default is True
cube.SetVisibility(True)
ring_slider.set_visibility(True)
hor_line_slider_text_top.set_visibility(True)
hor_line_slider_text_bottom.set_visibility(True)
ver_line_slider_text_left.set_visibility(True)
ver_line_slider_text_right.set_visibility(True)
Set camera for better visualization
show_manager.scene.reset_camera()
show_manager.scene.set_camera(position=(0, 0, 150))
show_manager.scene.reset_clipping_range()
show_manager.scene.azimuth(30)
interactive = False
if interactive:
show_manager.start()
fury.window.record(show_manager.scene, size=current_size, out_path="viz_slider.png")
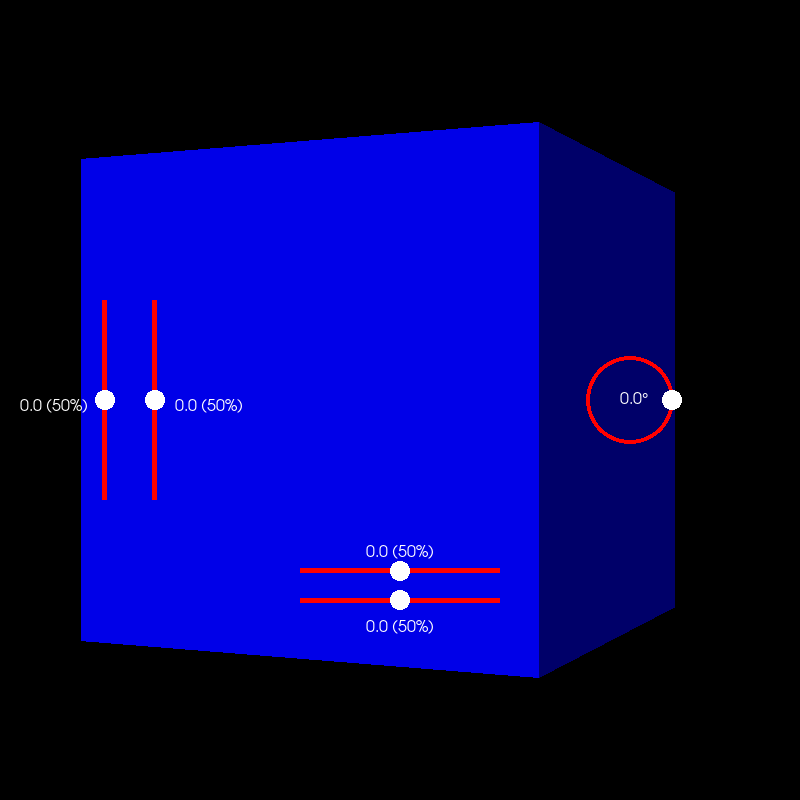
/opt/homebrew/Caskroom/miniforge/base/envs/py39/lib/python3.9/site-packages/sphinx_gallery/gen_rst.py:722: UserWarning: We'll no longer accept the way you call the record function in future versions of FURY.
Here's how to call the Function record: record(scene='value', cam_pos='value', cam_focal='value', cam_view='value', out_path='value', path_numbering='value', n_frames='value', az_ang='value', magnification='value', size='value', reset_camera='value', screen_clip='value', stereo='value', verbose='value')
exec(self.code, self.fake_main.__dict__)
Total running time of the script: (0 minutes 0.084 seconds)