Note
Click here to download the full example code
Visualize surfacesΒΆ
Here is a simple tutorial that shows how to visualize surfaces using DIPY. It
also shows how to load/save, get/set and update vtkPolyData
and show
surfaces.
vtkPolyData
is a structure used by VTK to represent surfaces and other data
structures. Here we show how to visualize a simple cube but the same idea
should apply for any surface.
import numpy as np
Import useful functions
from fury import window, utils
from fury.io import save_polydata, load_polydata
from fury.utils import vtk
Create an empty vtkPolyData
my_polydata = vtk.vtkPolyData()
Create a cube with vertices and triangles as numpy arrays
my_vertices = np.array([[0.0, 0.0, 0.0],
[0.0, 0.0, 1.0],
[0.0, 1.0, 0.0],
[0.0, 1.0, 1.0],
[1.0, 0.0, 0.0],
[1.0, 0.0, 1.0],
[1.0, 1.0, 0.0],
[1.0, 1.0, 1.0]])
# the data type for vtk is needed to mention here, numpy.int64
my_triangles = np.array([[0, 6, 4],
[0, 2, 6],
[0, 3, 2],
[0, 1, 3],
[2, 7, 6],
[2, 3, 7],
[4, 6, 7],
[4, 7, 5],
[0, 4, 5],
[0, 5, 1],
[1, 5, 7],
[1, 7, 3]], dtype='i8')
Set vertices and triangles in the vtkPolyData
Save the vtkPolyData
file_name = "my_cube.vtk"
save_polydata(my_polydata, file_name)
print("Surface saved in " + file_name)
Out:
Surface saved in my_cube.vtk
Load the vtkPolyData
add color based on vertices position
cube_vertices = utils.get_polydata_vertices(cube_polydata)
colors = cube_vertices * 255
utils.set_polydata_colors(cube_polydata, colors)
print("new surface colors")
print(utils.get_polydata_colors(cube_polydata))
Out:
new surface colors
[[ 0 0 0]
[ 0 0 255]
[ 0 255 0]
[ 0 255 255]
[255 0 0]
[255 0 255]
[255 255 0]
[255 255 255]]
Visualize surfaces
# get vtkActor
cube_actor = utils.get_actor_from_polydata(cube_polydata)
# Create a scene
scene = window.Scene()
scene.add(cube_actor)
scene.set_camera(position=(10, 5, 7), focal_point=(0.5, 0.5, 0.5))
scene.zoom(3)
# display
# window.show(scene, size=(600, 600), reset_camera=False)
window.record(scene, out_path='cube.png', size=(600, 600))
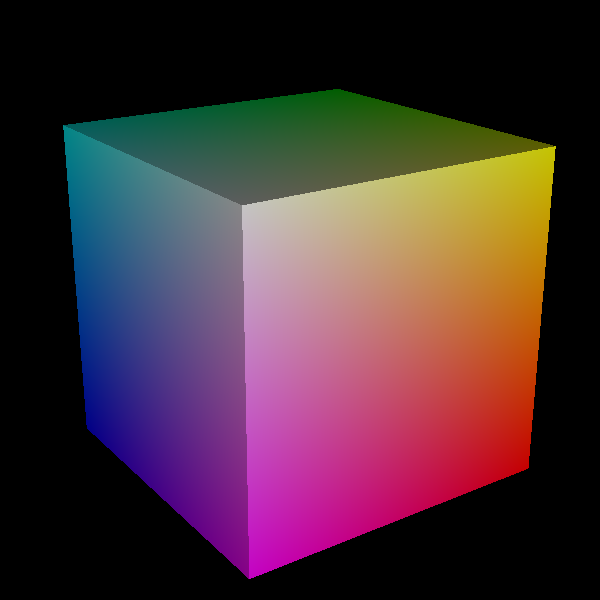
Total running time of the script: ( 0 minutes 0.105 seconds)